So just to kick off I thought I'd drop a little Cocoa code to apply a Core Image filter to, or underneath, a window. This is similar to what Apple themselves use in Mac OS X v10.5 "Leopard" to create a blur under menus, etc.
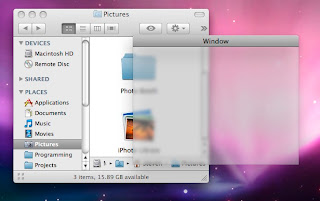
Firstly, you'll need to define some things:
typedef void * CGSConnection;
extern OSStatus CGSNewConnection(const void **attributes, CGSConnection * id);
Then you'll need to use the following code to create a filter and apply it to a window. For this example, I use a blur placed beneath a window's contents (similar to menus, or Glass in Vista):
-(void)enableBlurForWindow:(NSWindow *)window
{
CGSConnection thisConnection;
uint32_t compositingFilter;
int compositingType = 1; // Under the window
/* Make a new connection to CoreGraphics */
CGSNewConnection(NULL, &thisConnection);
/* Create a CoreImage filter and set it up */
CGSNewCIFilterByName(thisConnection, (CFStringRef)@"CIGaussianBlur", &compositingFilter);
NSDictionary *options = [NSDictionary dictionaryWithObject:[NSNumber numberWithFloat:3.0] forKey:@"inputRadius"];
CGSSetCIFilterValuesFromDictionary(thisConnection, compositingFilter, (CFDictionaryRef)options);
/* Now apply the filter to the window */
CGSAddWindowFilter(thisConnection, [window windowNumber], compositingFilter, compositingType);
}